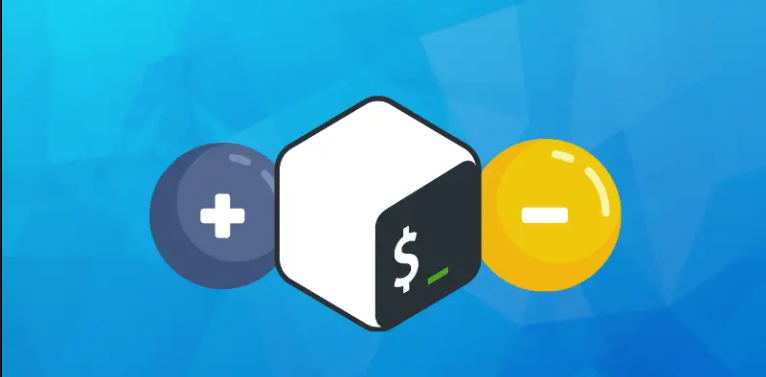
In the world of bash scripting, the ability to increment variables is a fundamental skill. Whether you’re tracking counts, iterating through loops, or updating values, knowing how to properly increment variables is crucial for writing robust and reliable shell scripts. This guide will explore the various techniques for incrementing variables in bash, covering both basic and advanced methods, as well as best practices and common pitfalls.
What is Bash Increment Variable
In bash, variables are used to store and manipulate data. Incrementing a variable involves increasing its value by a specified amount, usually by 1. This is a common operation in programming and scripting, and it’s particularly useful for tasks like counting, indexing, and tracking progress.
Basic Incrementing Methods
- Pre-increment:
counter=$((counter + 1))
This method first increments the variable and then assigns the new value back to the variable. - Post-increment:
((counter++))
This method assigns the current value of the variable and then increments it. - Shorthand increment:
((counter+=1))
This is a more compact way of incrementing a variable by 1.
Advanced Incrementing Techniques
- Incrementing by a specific value:
counter=$((counter + 5))
This increments the variable by 5 instead of the default 1. - Decrementing (subtracting) a variable:
counter=$((counter - 1))
This decreases the value of the variable by 1. - Compound assignment:
counter+=5
This increments the variable by 5 using the compound assignment operator. - Presets and resets:
counter=0 <em># Reset the counter to 0 </em>
counter=1 <em># Preset the counter to 1</em>
You can set the initial value of a variable before incrementing it.
Combining Increment with Other Operations
Incrementing and printing:
This increments the variable and then prints its value.
Incrementing and assigning to another variable:
This assigns the current value of the counter to the new_counter variable and then increments the original counter
Conditional incrementing:
This increments the counter only if it’s less than 10.
Best Practices for Incrementing Variables
Use meaningful variable names:
This makes the purpose of the variable clear.
Initialize variables before incrementing:
Ensure variables are properly initialized to avoid unexpected behavior.
Avoid ambiguous incrementation.
Be explicit about the type of incrementation you’re using.
Use the appropriate data type:
This ensures the variable is treated as an integer, preventing unintended behavior.
Handle overflow and underflow:
Consider the range of values the variable can hold and handle edge cases accordingly.
Common Pitfalls and Troubleshooting
Uninitialized variables:
This will result in an error or unexpected behavior.
Mixing pre- and post-increment:
Be consistent in the way you increment variables.
Floating-point arithmetic:
Use alternative tools like ‘bc’ for floating-point operations.
Overflow and underflow:
Monitor the range of your variables and handle edge cases appropriately.
Why Learn Bash Increment Variable?
Learning and mastering variable incrementation in bash is essential for a wide range of tasks, including:
- Tracking and updating counts
- Iterating through loops and arrays
- Generating unique identifiers
- Maintaining state in scripts
- Implementing progress indicators
By understanding the various techniques and best practices for incrementing variables, you can write more efficient, reliable, and maintainable shell scripts that can handle a variety of use cases.
Related Articles
https://linuxhandbook.com/bash-increment-decrement-counter
https://phoenixnap.com/kb/bash-increment-decrement-variable
More Articles from Unixmen