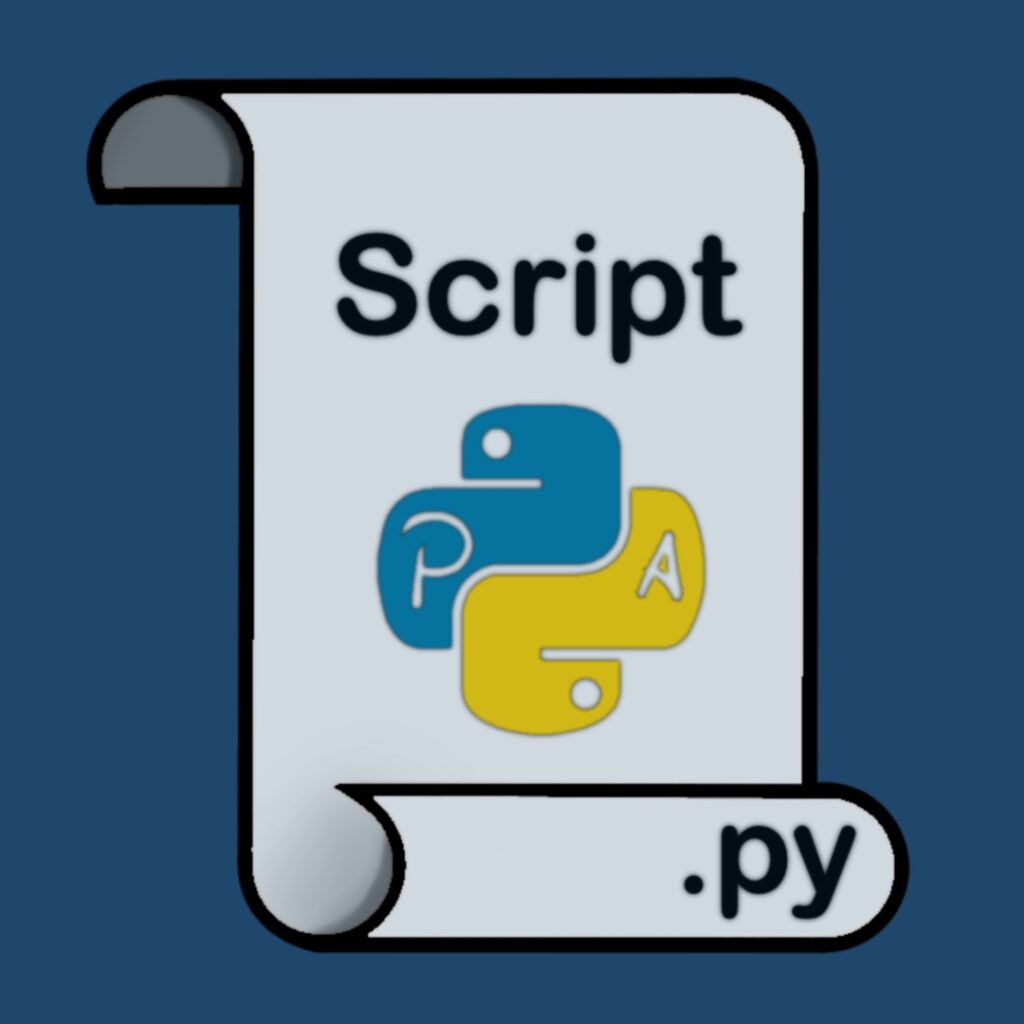
What is a Python Script?
A Python script is a file containing Python code that can be executed. These files typically have a `.py` extension and can range from simple one-liners to complex programs with multiple functions and modules. This comprehensive article will guide you in how to run python scripts effortlessly.
Prerequisites for Running Python Scripts
Before you can run a Python script, ensure you have:
- Python installed on your system (version 3.x recommended).
- A text editor or Integrated Development Environment (IDE).
- Basic knowledge of navigating your file system.
Running Python Scripts from the Command Line
Running Python scripts from the command line is a fundamental skill. Here’s how to do it:
- Open your terminal or command prompt
- Navigate to the directory containing your Python script
- Type `python` followed by the script name:
For Python 3, you might need to use `python3` instead:
How to Run a Python Script in an IDE
Many developers prefer using IDEs for a more integrated experience. Popular Python IDEs include PyCharm, Visual Studio Code, and IDLE. Here’s a general process:
- Open your IDE
- Open your Python script file
- Look for a “Run” or “Play” button, usually at the top of the IDE
- Click the button to execute your script
Most IDEs also offer keyboard shortcuts for running scripts, such as F5 in IDLE or Shift+F10 in PyCharm.
How to Run a Python Script on Different Operating Systems
Windows
- Open Command Prompt
- Navigate to your script’s directory
- Type `
python your_script.py
`
Alternatively, you can double-click the `.py` file if Python is correctly associated with `.py` extensions.
macOS and Linux
- Open Terminal
- Navigate to your script’s directory
- Type `
python3 your_script.py
`
You can also make your script executable:
- Add
`!/usr/bin/env python3`
as the first line of your script
- In the terminal, type `
chmod +x your_script.py`
- Run with `
./your_script.py
`
Common Issues and Troubleshooting When Running a Python Script
- “Python is not recognized as an internal or external command”
-The solution to this is to add Python to your system’s PATH environment variable
- “No such file or directory”
– In order to fix this ensure you’re in the correct directory and the file name is correct
- Import Error when using modules
This error occurs when the modules are not installed. You can install the modules using pip (`
`)
- Permission denied (on Unix-based systems)
– The solution is to use `
` to make the script executable
Best Practices for Running Python Scripts
- Use Virtual Environments: This keeps dependencies separate for different projects
- Comment Your Code: This helps others (and future you) understand your script
- Use Shebang Lines: On Unix-based systems, use
`!/usr/bin/env python3`
at the start of your script
- Error Handling: Implement try-except blocks to gracefully handle potential errors
- Input Validation: Always validate user inputs to prevent unexpected behavior
Advanced Techniques for Running Python Scripts
- Command-Line Arguments: Use `
sys.argv
` or the `
argparse` module to pass arguments to your script.
- Scheduling Scripts: Use tools like cron (Unix) or Task Scheduler (Windows) to run scripts automatically.
- Running as a Service: For long-running scripts, consider setting them up as a system service.
- Python’s
`-m`
Flag: Run modules as scripts using `
python -m module_name`.
- IPython and Jupyter Notebooks: For interactive development and data analysis.
By trying out these techniques, you’ll be able to efficiently run Python scripts in different environments. Remember, practice makes perfect – the more you work with Python, the more comfortable you’ll become with these processes.
More Articles from Unixmen
Here are a few Python coding tips for every beginner coding geek