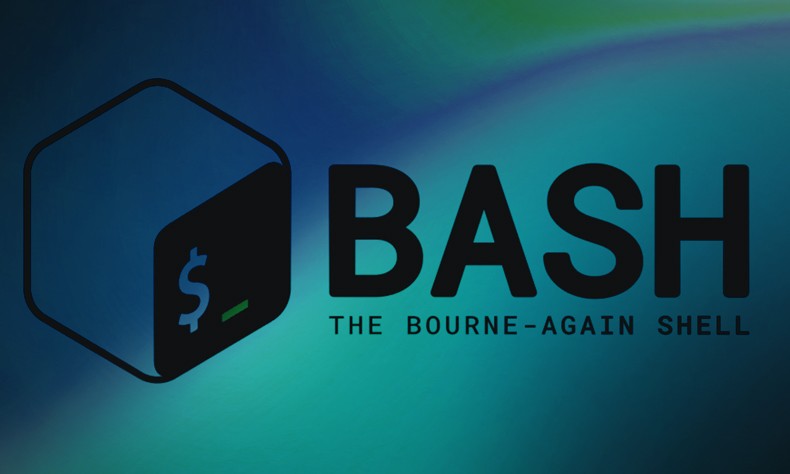
Bash scripting is a powerful tool for automating tasks in Unix-based systems, including Linux and macOS. Whether you’re a system administrator, developer, or enthusiast, understanding bash scripts can significantly enhance your productivity. This guide will walk you through a practical bash script example, breaking down its components and explaining key concepts along the way.
What is a Bash Script?
A bash script is a text file containing a series of commands that the bash shell can execute. It allows you to automate repetitive tasks, create custom commands, and build complex workflows.
Basic Structure of a Bash Script
- Shebang line:
#!/bin/bash
- Comments:
# This is a comment
- Variables:
NAME="John"
- Commands:
echo "Hello, $NAME"
- Control structures: if statements, loops, etc.
A Practical Bash Script Example Let’s create a script that performs system maintenance tasks
Breaking Down the Script
- Shebang line:
This line tells the system to use the bash interpreter.#!/bin/bash
- Comments:
Script Comments help explain the script’s purpose and functionality.# System Maintenance
- Echo statements:
These provide user feedback during script execution.echo "Updating package lists..."
- System commands:
-
Updates the package listssudo apt update:
-
Upgrades installed packagessudo apt upgrade -y:
-
Removes unused packagessudo apt autoremove -y:
-
Clears the apt cachesudo apt clean:
-
Displays disk usagedf -h:
-
Shows system uptimeuptime:
-
-
The script uses sudo for commands that require elevated privileges.Sudo usage:
Running the Script
- Save the script as
"system_maintenance.sh"
- Make it executable:
chmod +x system_maintenance.sh
- Run the script:
./system_maintenance.sh
Common Bash Scripting Concepts
- Variables
- Conditional Statements
- Loops
- Functions
- Command Substitution
Best Practices for Bash Scripting
- Always use the shebang line:
#!/bin/bash
- Comment your code for clarity
- Use meaningful variable names
- Quote variables to prevent word splitting
- Use exit codes to indicate script success or failure
- Handle errors and edge cases
- Use shellcheck to validate your scripts
Troubleshooting Common Issues
- Permission denied: Ensure the script is executable
(chmod +x)
- Command not found: Check the path to the command or use full paths
- Unexpected behavior: Use set -x for debugging
- Syntax errors: Validate your script with shellcheck
Why You Should Learn Bash Scripting.
Bash scripting is a valuable skill for anyone working with Unix-based systems. It allows you to:
- Automate repetitive tasks
- Create custom system administration tools
- Enhance productivity and efficiency
- Perform complex operations with simple commands
This bash script example demonstrates how a simple script can automate several system maintenance tasks. By understanding the structure and components of this script, you can start creating your own bash scripts to automate various aspects of your work. Remember to follow best practices, comment your code, and continuously learn new bash features to improve your scripting skills.
Related Articles
Bash Scripting Tutorial – Linux Shell Script and Command
25 Common Linux Bash Script Examples to Get You Started
More Articles from Unixmen