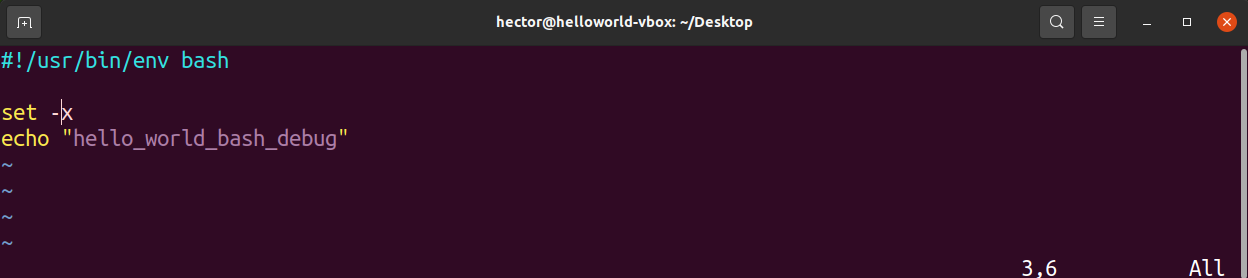
The shebang, also known as ‘hashbang’ or pound-bang, is a crucial element in Unix-like operating systems, including Linux. It’s the first line of a script that tells the system which interpreter to use when executing the file. Understanding the bash shebang is fundamental for anyone writing shell scripts or working with Unix-based systems. This guide will explore the concept, syntax, and best practices for using shebangs in your bash scripts.
So What is a Shebang?
A shebang is a special character sequence at the beginning of a script file that specifies which interpreter should be used to execute the script. It consists of a hash symbol (#) followed by an exclamation mark (!), hence the name “shebang.”
Basic Bash Shebang Syntax
The basic syntax for a bash shebang is:
This line informs the system to use the bash interpreter located at /bin/bash to execute the script.
Why You Should Integrate Shebang in Your Scripts?
- Interpreter specification: Shebang is commonly used to clearly define which interpreter to use
- Execution flexibility: The command also allows scripts to be run directly, without specifying the interpreter
- Improved portability: Helps scripts work across different systems
- Enhanced clarity: Immediately indicates the script type to readers
Common Bash Shebang Variations
- Bash:
#!/bin/bash - Sh (POSIX-compliant shell):
#!/bin/sh - Python:
#!/usr/bin/env python3 - Perl:
#!/usr/bin/perl - Ruby:
#!/usr/bin/env ruby
Best Practices for Using Bash Shebang
- It is recommended to always include a shebang in executable scripts
- Use the full path to the interpreter
- Make your scripts executable with chmod +x
- Consider using /usr/bin/env for improved portability
- Choose the appropriate interpreter for your script’s needs
- Place the shebang on the very first line of the file
Shebang Portability Issues
- Path differences: Interpreter locations may vary across systems
- Limited path length: Some systems have restrictions on shebang line length
- Interpreter availability: The specified interpreter may not be installed on all systems
To address these issues, you should consider using the following approach:
This method uses the env command to locate the bash interpreter, improving portability across different Unix-like systems.
Advanced Bash Shebang Techniques
- When using multiple interpreters:
#!/bin/bash<br />#!/bin/sh
Note: Only the first shebang is used; others are treated as comments. - Passing options to the interpreter:
#!/bin/bash -x
This enables debug mode for the script. - Using specific interpreter versions:
#!/usr/bin/env python3.8 - Shebang with parameters:
#!/usr/bin/env -S bash -O extglob
This uses env’s -S option to pass multiple arguments.
Troubleshooting Common Shebang Problems
- “Bad interpreter” error: • Check if the specified interpreter exists and is accessible • Ensure the script has correct line endings (Unix-style LF, not Windows CRLF)
- Script not executing: • Verify that the script is executable (chmod +x script.sh) • Check for typos in the shebang line
- Unexpected behavior: • Confirm you’re using the intended interpreter • Check for system-specific quirks or limitations
- Portability issues: • Consider using /usr/bin/env for better cross-system compatibility • Test your script on different systems to ensure compatibility
Why is Shebang Important
Understanding and properly using shebangs is crucial for several reasons:
- Script execution: Ensures scripts run with the correct interpreter
- Portability: Improves script functionality across different systems
- Clarity: Immediately indicates the script type and requirements
- Flexibility: Allows for easy switching between interpreters
- Professionalism: Demonstrates good scripting practices
While bash shebang might seem like a small component in shell scripting, understanding its purpose, syntax, you can create more effective and portable scripts. Remember to always include an appropriate shebang in your executable scripts, consider portability issues, and choose the right interpreter for your needs.
Related Articles
https://linuxize.com/post/bash-shebang
https://phoenixnap.com/kb/shebang-bash
More Articles from Unixmen